Build a Remote MCP Server with Node-RED
Now that Node-RED is up and running, let’s create your first flow—a simple HTTP GET endpoint that registers a session and enables communication between your Remote MCP Server and clients (such as AI agents).
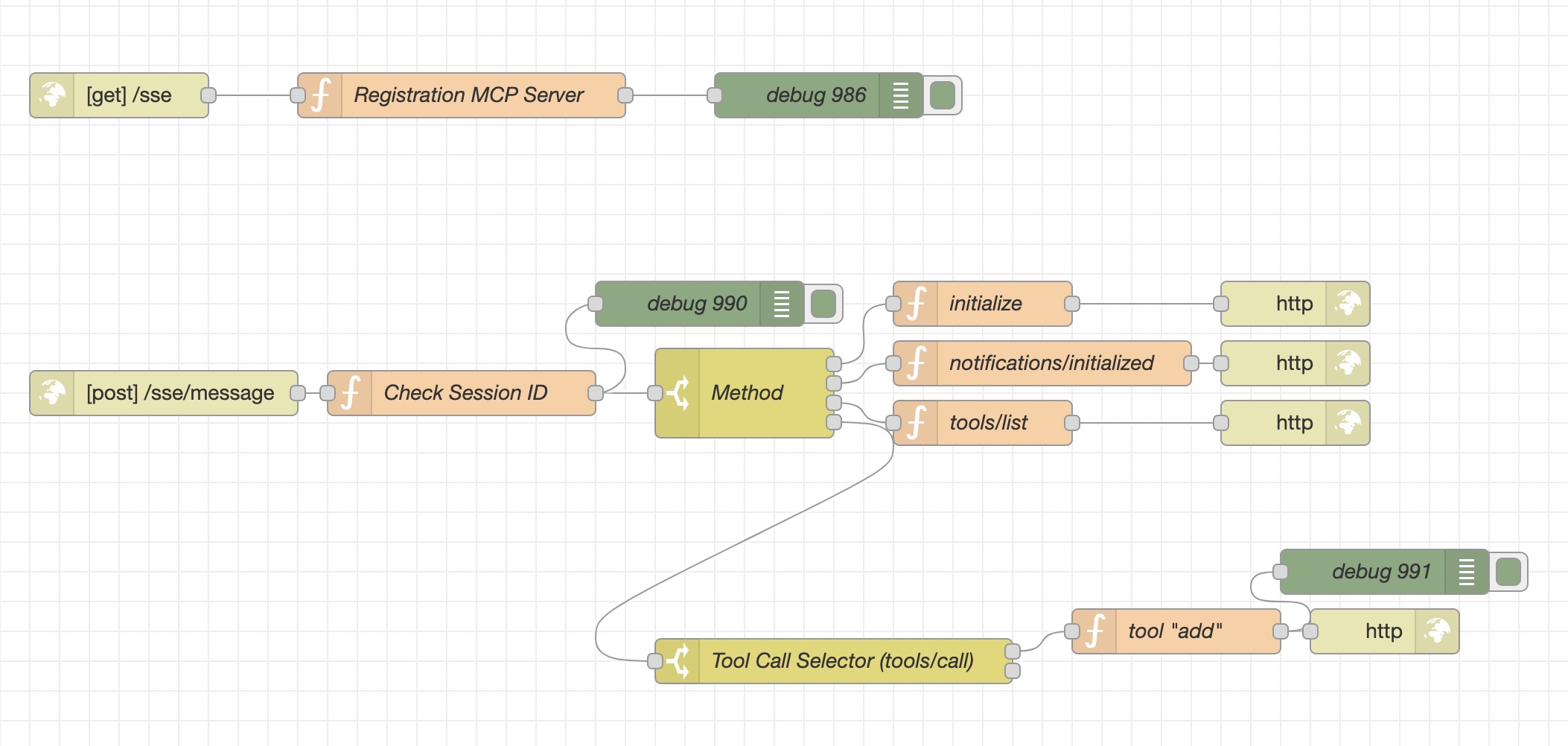
The MCP Server uses several HTTP endpoints to handle incoming requests and manage communication:
- GET /sse: This endpoint is used to establish a Server-Sent Events (SSE) connection. It initializes a session and sends endpoint information to the client.
- POST /sse/message: This endpoint receives messages from clients and processes them based on the session ID.
Each endpoint is configured using Node-RED's HTTP nodes, which allow for easy setup and management of HTTP requests.
Step 1: [GET] /sse endpoint

This flow sets up a Server-Sent Events (SSE) endpoint, allowing clients to connect and receive real-time updates. The core logic happens inside a Function node, which:
- Generates a unique session ID.
- Stores session info in memory.
- Configures response headers for SSE.
- Sends a first message with the client-facing endpoint.
- Starts polling for messages every second.
The flow consists of these components:
- HTTP In Node – Listens for GET requests on
/sse
. - Function Node – Handles session logic, header configuration, and polling.
- Debug Node – Outputs messages to the debug sidebar for development visibility.
Function Node Breakdown: Server Registration Logic
function generateUUID() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) {
const r = Math.random() * 16 | 0,
v = c === 'x' ? r : (r & 0x3 | 0x8);
return v.toString(16);
});
}
const sessionId = generateUUID();
// Store session (optional)
let sessions = global.get("sse_sessions") || {};
sessions[sessionId] = {
createdAt: new Date().toISOString(),
sseRes: msg.res._res
};
global.set("sse_sessions", sessions);
// Set headers
msg.res._res.setHeader("Content-Type", "text/event-stream");
msg.res._res.setHeader("Cache-Control", "no-cache");
msg.res._res.setHeader("Connection", "keep-alive");
msg.res._res.setHeader("access-control-max-age", "86400");
msg.res._res.setHeader("Access-Control-Allow-Origin", "*");
msg.res._res.setHeader("Access-Control-Allow-Methods", "GET, POST, OPTIONS");
msg.res._res.setHeader("Access-Control-Allow-Headers", "Authorization, *");
// Compose payload
msg.endpoint = "/sse/message?sessionId=" + sessionId;
// Send initial SSE message with endpoint info
msg.res._res.write(`event: endpoint\ndata: ${msg.endpoint}\n\n`);
//Periodic Message Polling and Sending
const messagePoller = setInterval(() => {
const currentSessions = global.get("sse_sessions") || {};
const session = currentSessions[sessionId];
if (!session) {
clearInterval(messagePoller);
return;
}
session.messages = session.messages || [];
for (let i = 0; i < session.messages.length; i++) {
const msgObj = session.messages[i];
if (!msgObj.sent) {
session.sseRes.write(`event: message\ndata: ${JSON.stringify(msgObj.data)}\n\n`);
msgObj.sent = true;
}
}
// Save session state
currentSessions[sessionId] = session;
global.set("sse_sessions", currentSessions);
}, 1000);
return null;
This function
node handles the core logic when a client connects to the /sse
endpoint. Here's a step-by-step explanation:
function generateUUID() { ... }
: Generates a unique identifier (sessionId
) for the connecting client using a standard UUID format.const sessionId = generateUUID();
: Calls thegenerateUUID()
function to create a unique ID for the current session.Session Management:
let sessions = global.get("sse_sessions") || {};
: Retrieves the global objectsse_sessions
(or initializes it if it doesn't exist). This object stores information about active client connections.sessions[sessionId] = { createdAt: new Date().toISOString(), sseRes: msg.res._res };
: Creates a new entry in thesessions
object for the currentsessionId
. It stores the connection timestamp and the raw HTTP response object (msg.res._res
), which is essential for sending SSE events.global.set("sse_sessions", sessions);
: Updates thesse_sessions
object in the global context, making the session information persistent.
SSE Header Setup:
msg.res._res.setHeader("Content-Type", "text/event-stream");
: Sets the crucial header to indicate that the server will send a stream of events.msg.res._res.setHeader("Cache-Control", "no-cache");
: Prevents client-side caching of the event stream.msg.res._res.setHeader("Connection", "keep-alive");
: Keeps the HTTP connection open for continuous event streaming.msg.res._res.setHeader("Access-Control-Allow-Origin", "*");
: Configures CORS to allow connections from any origin (can be restricted for production).
Message Endpoint Definition:
msg.endpoint = "/sse/message?sessionId=" + sessionId;
: Creates a URL (/sse/message
) with the uniquesessionId
as a parameter. This is the endpoint the client will use to send messages back to the server. This endpoint is stored in themsg
object for later use.
Initial SSE Event:
msg.res._res.write(\
event: endpoint\ndata: ${msg.endpoint}\n\n`);`: Sends the first Server-Sent Event to the client. Theevent
type isendpoint
, and thedata
contains the message endpoint URL created in the previous step. The double newline (\n\n
) signifies the end of the SSE event.
Periodic Message Handling:
const messagePoller = setInterval(() => { ... }, 1000);
: Sets up an interval to run a function every 1000 milliseconds (1 second). This function checks for and sends any buffered messages to the client.const currentSessions = global.get("sse_sessions") || {};
: Retrieves the current session information.const session = currentSessions[sessionId];
: Gets the specific session for the current client.if (!session) { clearInterval(messagePoller); return; }
: If the session no longer exists, the interval is cleared to prevent errors.session.messages = session.messages || [];
: Ensures thesession
object has amessages
array (likely populated elsewhere with messages to send).for (let i = 0; i < session.messages.length; i++) { ... }
: Iterates through themessages
array.if (!msgObj.sent) { ... }
: Checks if a message has already been sent.session.sseRes.write(\
event: message\ndata: ${JSON.stringify(msgObj.data)}\n\n`);`: Sends the message as an SSE event of typemessage
. The message data is stringified as JSON.msgObj.sent = true;
: Marks the message as sent.global.set("sse_sessions", currentSessions);
: Updates the globalsse_sessions
with the potentially modified session data.
return null;
: The function does not pass any message to the next node in the flow, as its primary purpose is to manage the HTTP response and global state for the SSE connection.
Full Flow JSON of MCP Registration Endpoint
[
{
"id": "c882531a6a2da6a2",
"type": "http in",
"z": "3321740562069c7c",
"name": "",
"url": "/sse",
"method": "get",
"upload": false,
"swaggerDoc": "",
"x": 100,
"y": 60,
"wires": [
[
"2a76a8cf4603effc",
"2f4cbc634ee2512c"
]
]
},
{
"id": "2a76a8cf4603effc",
"type": "debug",
"z": "3321740562069c7c",
"name": "debug 986",
"active": true,
"tosidebar": true,
"console": false,
"tostatus": false,
"complete": "true",
"targetType": "full",
"statusVal": "",
"statusType": "auto",
"x": 490,
"y": 60,
"wires": []
},
{
"id": "2f4cbc634ee2512c",
"type": "function",
"z": "3321740562069c7c",
"name": "function 877",
"func": "function generateUUID() {\n return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) {\n const r = Math.random() * 16 | 0,\n v = c === 'x' ? r : (r & 0x3 | 0x8);\n return v.toString(16);\n });\n}\n\nconst sessionId = generateUUID();\n\n// Store session (optional)\nlet sessions = global.get(\"sse_sessions\") || {};\nsessions[sessionId] = {\n createdAt: new Date().toISOString(),\n sseRes: msg.res._res\n};\nglobal.set(\"sse_sessions\", sessions);\n\n// Set headers\nmsg.res._res.setHeader(\"Content-Type\", \"text/event-stream\");\nmsg.res._res.setHeader(\"Cache-Control\", \"no-cache\");\nmsg.res._res.setHeader(\"Connection\", \"keep-alive\");\nmsg.res._res.setHeader(\"access-control-max-age\", \"86400\");\nmsg.res._res.setHeader(\"Access-Control-Allow-Origin\", \"*\");\nmsg.res._res.setHeader(\"Access-Control-Allow-Methods\", \"GET, POST, OPTIONS\");\nmsg.res._res.setHeader(\"Access-Control-Allow-Headers\", \"Authorization, *\");\n\n// Compose payload\nmsg.endpoint = \"/sse/message?sessionId=\" + sessionId;\n\n// Send initial SSE message with endpoint info\nmsg.res._res.write(`event: endpoint\\ndata: ${msg.endpoint}\\n\\n`);\n\nconst messagePoller = setInterval(() => {\n const currentSessions = global.get(\"sse_sessions\") || {};\n const session = currentSessions[sessionId];\n\n if (!session) {\n clearInterval(messagePoller);\n return;\n }\n\n session.messages = session.messages || [];\n\n for (let i = 0; i < session.messages.length; i++) {\n const msgObj = session.messages[i];\n if (!msgObj.sent) {\n session.sseRes.write(`event: message\\ndata: ${JSON.stringify(msgObj.data)}\\n\\n`);\n msgObj.sent = true;\n }\n }\n\n // Save session state\n currentSessions[sessionId] = session;\n global.set(\"sse_sessions\", currentSessions);\n}, 1000);\n\n\nreturn null;\n",
"outputs": 1,
"timeout": 0,
"noerr": 0,
"initialize": "",
"finalize": "",
"libs": [],
"x": 290,
"y": 60,
"wires": [
[
"2a76a8cf4603effc"
]
]
}
]
Debugging Setup
Debugging is an essential part of the MCP Server implementation. Node-RED provides debug nodes that can be used to log messages and monitor the flow of data:
- Debug Nodes: These nodes are connected to various points in the flow to output messages to the Node-RED debug sidebar. This helps in tracking the flow of data and identifying any issues.
By understanding the HTTP endpoints and debugging setup, you can effectively manage and troubleshoot the MCP Server. In the next step, we'll delve into session management and SSE implementation.
Debugging Server Registration with MCP Inspector
To effectively test and debug the server registration process of your Remote MCP Server, we'll utilize the MCP Inspector, an open-source developer tool specifically designed for interacting with MCP servers.
The MCP Inspector allows you to send requests to your server and inspect the responses, making it invaluable for verifying that your registration endpoint is working as expected.
Installation and Basic Usage
The great thing about the MCP Inspector is that it runs directly through npx
(Node Package Runner), which comes bundled with Node.js. This means you don't need to install it globally!
To use the Inspector, simply open your terminal or command prompt and run the following command:
npx @modelcontextprotocol/inspector <command>
After running this command, you should see the MCP Inspector interface appear.
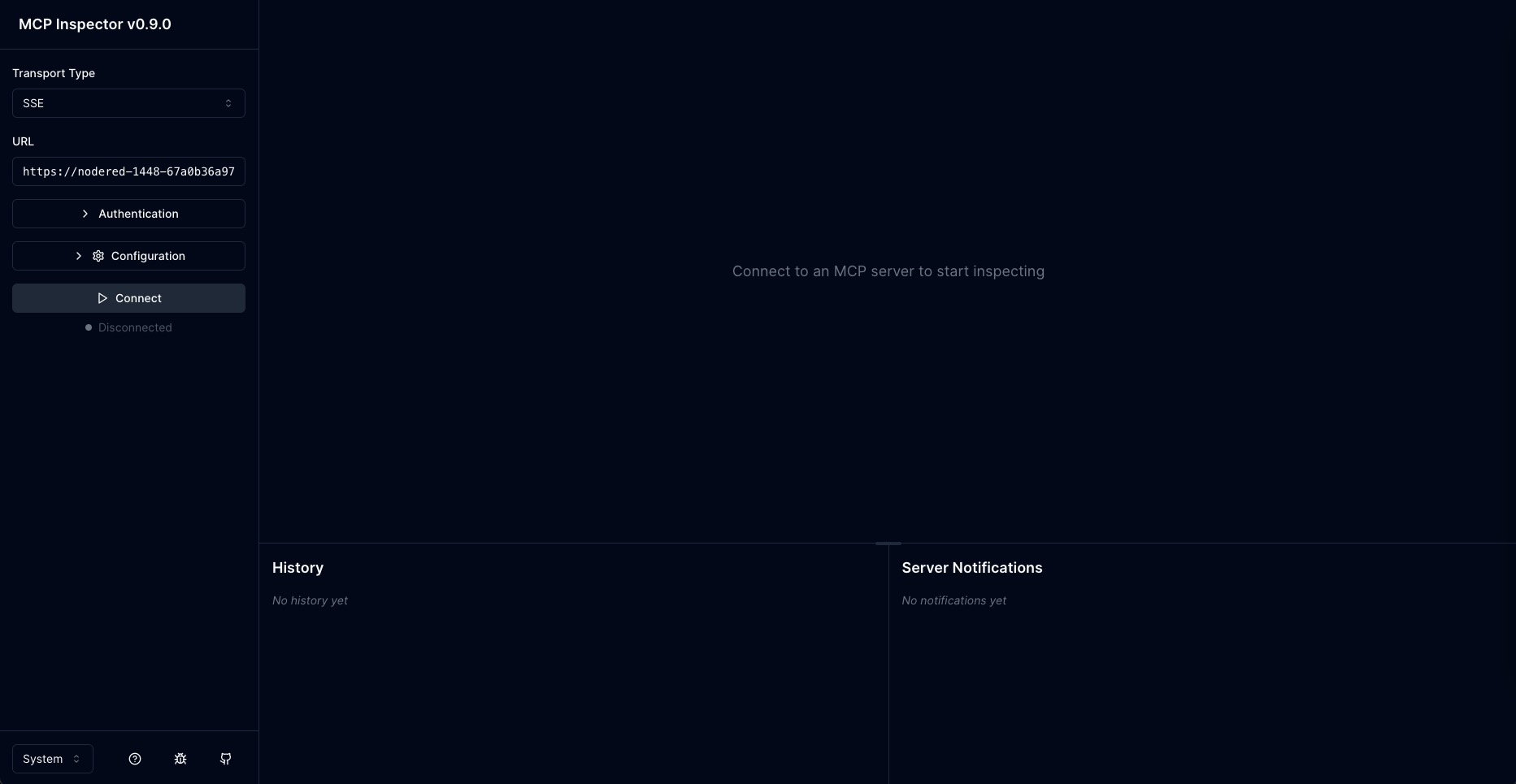
Connecting to Your Node-RED Server
To test the server registration endpoint, follow these steps in the MCP Inspector:
Transport Type: In the MCP Inspector interface, locate the "Transport Type" dropdown menu and select "SSE" (Server-Sent Events).
URL: In the "URL" field, enter the full URL to your Node-RED
/sse
endpoint. This will be the address where your Node-RED server is running, followed by/sse
. For example:https://your-node-red-url.ubos.tech/sse
Replace
https://your-node-red-url.ubos.tech
with the actual URL of your Node-RED instance.
Once you have selected "SSE" as the transport type and entered the correct URL to your /sse
endpoint, you can proceed to connect and inspect the communication. The next steps will involve initiating the connection and examining the server's response in the MCP Inspector.
- Click "Connect": Look for the "Connect" button in the MCP Inspector interface and click it.
Upon clicking "Connect", the MCP Inspector will send a GET request to your Node-RED server's `/sse` endpoint. If your Node-RED flow is correctly deployed, you should observe the following:
- Node-RED Debug Output: In your Node-RED debug sidebar, you should see the
msg
object that was received by thehttp in
node. This will contain information about the incoming request from the MCP Inspector.
This confirms that the MCP Inspector is successfully reaching your Node-RED server and triggering the /sse
endpoint. In the subsequent steps, we will examine the response received by the MCP Inspector to ensure that the server registration process is functioning as expected.
Step 2: Handling Incoming Messages via POST /sse/message
Now that we have established the initial SSE connection, we need to create a new flow to handle messages sent from the client (typically an AI agent) to our MCP server. This will be done via a POST
request to the /sse/message
endpoint.
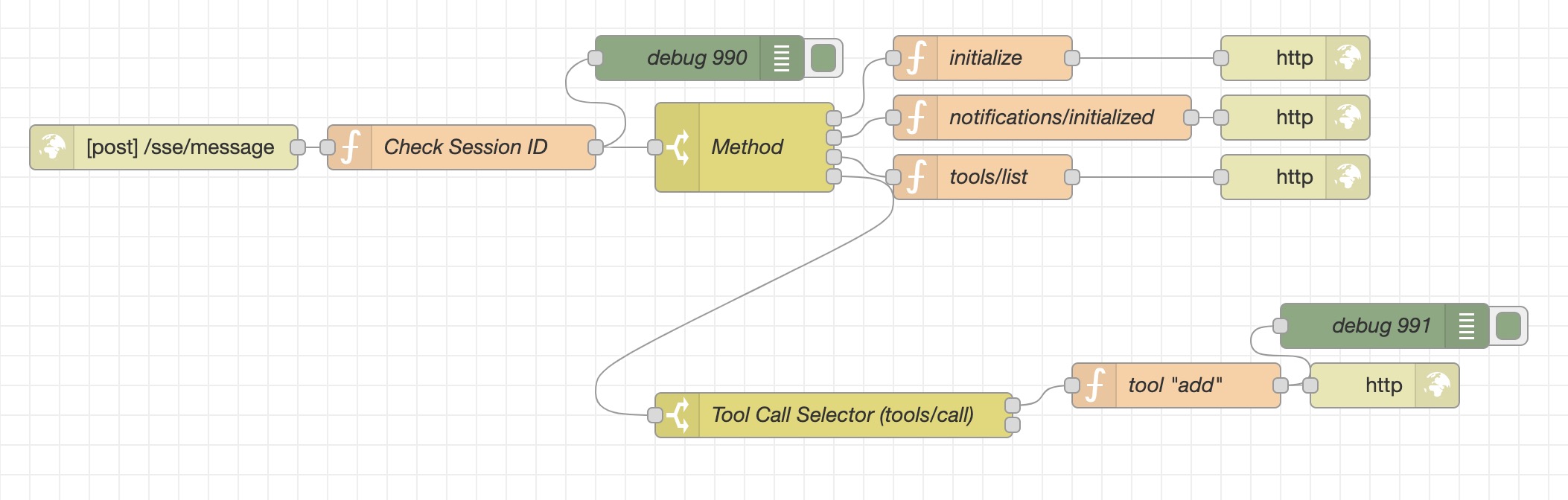
http in
Node (POST /sse/message)
This node acts as the entry point for all incoming HTTP POST
requests directed to the /sse/message
URL on your Node-RED server.
- Type:
http in
- Method:
POST
- This signifies that this endpoint expects to receive data from the client, usually within the body of the request. In the context of MCP, this is where commands and associated information from the AI agent will be sent. - URL:
/sse/message
- This is the specific path on your Node-RED server that clients will target when sending messages. To correctly associate the message with a specific SSE session, the client will also include thesessionId
(obtained during the initial/sse
connection) as a query parameter in this URL (e.g.,/sse/message?sessionId=your-unique-session-id
).
function
Node (Check Session ID)
This function
node is crucial for validating that the incoming message originates from a currently active and registered client session.
let sessions = global.get("sse_sessions") || {};
const sessionId = msg.req.query.sessionId;
if (!sessions[sessionId]) {
node.warn(`No active SSE stream for session: ${sessionId}`);
return null;
}
return msg;
let sessions = global.get("sse_sessions") || {};
:- Retrieves the
sse_sessions
object from Node-RED's global context, where active SSE sessions are stored. - If
sse_sessions
doesn't exist, it initializessessions
as an empty object to prevent errors.
- Retrieves the
const sessionId = msg.req.query.sessionId;
:- Extracts the value of the
sessionId
query parameter from the incoming HTTP request's URL. ThissessionId
should have been provided by the client during the initial SSE connection.
- Extracts the value of the
if (!sessions[sessionId]) { ... }
:- Checks if a session with the extracted
sessionId
exists as a key in thesessions
object. If not (meaning thesessionId
is invalid or the session has expired), the code inside theif
block is executed. node.warn(\
No active SSE stream for session: ${sessionId}`);`: Logs a warning message to the Node-RED console indicating that a message was received for an inactive session.return null;
: Stops the processing of the current message (msg
) in the flow, as it's not associated with a valid active session.
- Checks if a session with the extracted
return msg;
:- If the
sessionId
is found in thesessions
object (meaning the session is active), this line returns the originalmsg
object. This allows the message to proceed to the next nodes in the flow for further processing based on the message content.
- If the
Routing Initial MCP Client Methods
The first switch
node in our /sse/message
handling flow plays a crucial role in directing incoming requests based on the method
specified in the MCP client's JSON payload. This allows us to handle different initial commands sent by the client in a structured way. This section focuses on the processing of the first three common initial methods: initialize
, notifications/initialized
, and tools/list
. The fourth method, tools/call
, which involves invoking specific server-side functionalities, will be explained in more detail in a subsequent section.
- The
switch
node is configured with rules to match the following MCP methods:"initialize"
"notifications/initialized"
"tools/list"
"tools/call"
Handling initialize
Method
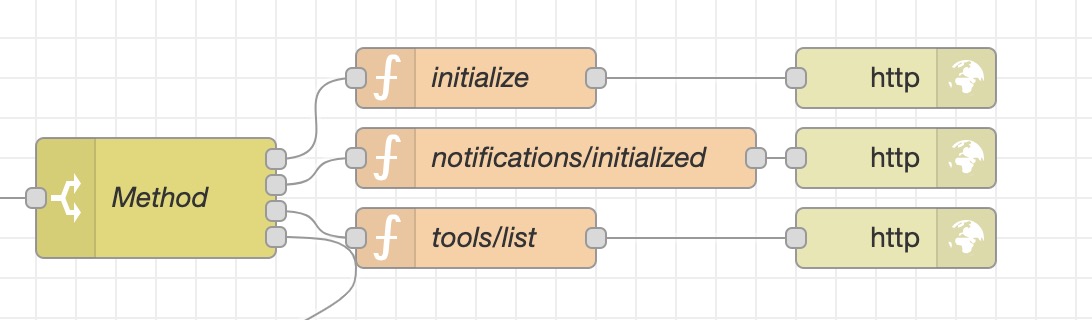
When the MCP client sends a
POST
request to/sse/message
with"method": "initialize"
in its payload, thisswitch
node routes themsg
object to thefunction
node named "initialize".The "initialize" function then processes this request by:
- Retrieving the current session information.
- Constructing a JSON response that includes details about the MCP server, such as the supported protocol version, server capabilities (initially empty for tools in this example), and server identification (name and version).
- Queuing this server information to be sent back to the client as an SSE
message
event. - Responding to the initial
POST
request with an HTTP "Accepted" status.
MCP Client Input Example:
{
"jsonrpc": "2.0",
"id": 0,
"method": "initialize",
"params": {
"protocolVersion": "2024-11-05",
"capabilities": {
"sampling": {},
"roots": {
"listChanged": true
}
},
"clientInfo": {
"name": "mcp-inspector",
"version": "0.9.0"
}
}
}MCP Server Output Example:
{
"result": {
"protocolVersion": "2024-11-05",
"capabilities": {
"tools": {}
},
"serverInfo": {
"name": "Demo",
"version": "1.0.0"
}
},
"jsonrpc": "2.0",
"id": 0
}Code Explanation for "initialize" Function:
let sessions = global.get("sse_sessions") || {};
let session = sessions[msg.req.query.sessionId];
if (session) {
session.messages = session.messages || [];
msg.info = { "result": { "protocolVersion": "2024-11-05", "capabilities": { "tools": {} }, "serverInfo": { "name": "Demo", "version": "1.0.0" } }, "jsonrpc": "2.0", "id": session.messages.length }
session.messages.push({
data: msg.info, // message to send
sent: false // initially unsent
});
global.set("sse_sessions", sessions);
}
msg.payload = "Accepted"
msg.res._res.setHeader("Content-Type", "text/plain");
return msg;let sessions = global.get("sse_sessions") || {};
: Retrieves the globalsse_sessions
object.let session = sessions[msg.req.query.sessionId];
: Gets the specific session object using thesessionId
from the request's query parameters.if (session) { ... }
: Checks if the session exists.session.messages = session.messages || [];
: Initializes thesession.messages
array if it doesn't exist. This array will hold messages to be sent to the client.msg.info = { ... }
: Constructs the JSON response containing the MCP server's information (protocol version, capabilities, server name, and version). Theid
is set to the current number of messages in the session's queue.session.messages.push({ data: msg.info, sent: false });
: Adds the constructed response to thesession.messages
array with asent
flag set tofalse
, indicating it hasn't been sent to the client yet.global.set("sse_sessions", sessions);
: Updates the globalsse_sessions
object with the new message in the queue for this session.
msg.payload = "Accepted"
: Sets the payload for the HTTP response.msg.res._res.setHeader("Content-Type", "text/plain");
: Sets the Content-Type header for the HTTP response.return msg;
: Returns themsg
object, which will then be used by thehttp response
node to send a "200 OK" with the "Accepted" text back to the client for the initialPOST
request. The actual server information is sent asynchronously via the SSE connection.
Handling notifications/initialized
Method
Upon receiving a
POST
request with"method": "notifications/initialized"
, theswitch
node directs themsg
to thefunction
node named "notifications/initialized".The "notifications/initialized" function, in the current implementation, primarily acknowledges the receipt of this notification by sending an HTTP "Accepted" response back to the client. This method signals that the client has processed the server's initial information and is ready for further interaction. While the current flow doesn't immediately send an SSE event back for this notification, you could extend this function to perform server-side actions upon receiving this confirmation.
Code Explanation for "notifications/initialized" Function:
msg.payload = "Accepted"
msg.res._res.setHeader("Content-Type", "text/plain");
return msg;- This function simply sets the payload to "Accepted" and sets the
Content-Type
header for the HTTP response. - It then returns the
msg
object, resulting in an HTTP "200 OK" with the "Accepted" text being sent back to the client. This acknowledges that the server has received the client's notification. No immediate SSE event is sent back in this basic implementation.
- This function simply sets the payload to "Accepted" and sets the
Handling tools/list
Method
When the MCP client sends a request with
"method": "tools/list"
, theswitch
node routes themsg
to thefunction
node named "tools/list".The "tools/list" function processes this request by:
- Retrieving the current session.
- Generating a JSON response that lists the tools available on the MCP server. This includes the name of each tool and its
inputSchema
, which describes the expected format and types of arguments for invoking the tool. The example flow provides a single tool named "add" with a schema defining two required number parameters, "a" and "b". - Queuing this list of tools to be sent back to the client as an SSE
message
event. - Responding to the initial
POST
request with an HTTP "Accepted" status.
MCP Client Input Example:
{
"method": "tools/list",
"jsonrpc": "2.0",
"id": 1
}MCP Server Output Example:
{
"result": {
"tools": [
{
"name": "add",
"inputSchema": {
"type": "object",
"properties": {
"a": {
"type": "number"
},
"b": {
"type": "number"
}
},
"required": [
"a",
"b"
],
"additionalProperties": false,
"$schema": "[http://json-schema.org/draft-07/schema#](http://json-schema.org/draft-07/schema#)"
}
}
]
},
"jsonrpc": "2.0",
"id": 0
}Code Explanation for "tools/list" Function:
let sessions = global.get("sse_sessions") || {};
let session = sessions[msg.req.query.sessionId];
if (session) {
session.messages = session.messages || [];
msg.tools = { "result": { "tools": [{ "name": "add", "inputSchema": { "type": "object", "properties": { "a": { "type": "number" }, "b": { "type": "number" } }, "required": ["a", "b"], "additionalProperties": false, "$schema": "[http://json-schema.org/draft-07/schema#](http://json-schema.org/draft-07/schema#)" } }] }, "jsonrpc": "2.0", "id": session.messages.length }
session.messages.push({
data: msg.tools, // message to send
sent: false // initially unsent
});
global.set("sse_sessions", sessions);
}
msg.payload = "Accepted"
msg.res._res.setHeader("Content-Type", "text/plain");
return msg;- Retrieves the global
sse_sessions
object and the specific session. - Constructs the JSON response containing the list of available tools (in this case, "add" with its schema). The
id
is set to the current number of messages in the session's queue. - Queues this list of tools to be sent back to the client as an SSE
message
event. - Responds to the initial
POST
request with "Accepted".
- Retrieves the global
Handling the tools/call
Method
The first switch
node ("Method") also handles the "tools/call"
method, routing it to a subsequent part of the flow involving the second switch
node ("Tool Call Selector (tools/call)") and dedicated function
nodes for each tool. This more complex process will be detailed in the next section of this documentation.
Handling tools/call
Method: Invoking Server-Side Tools
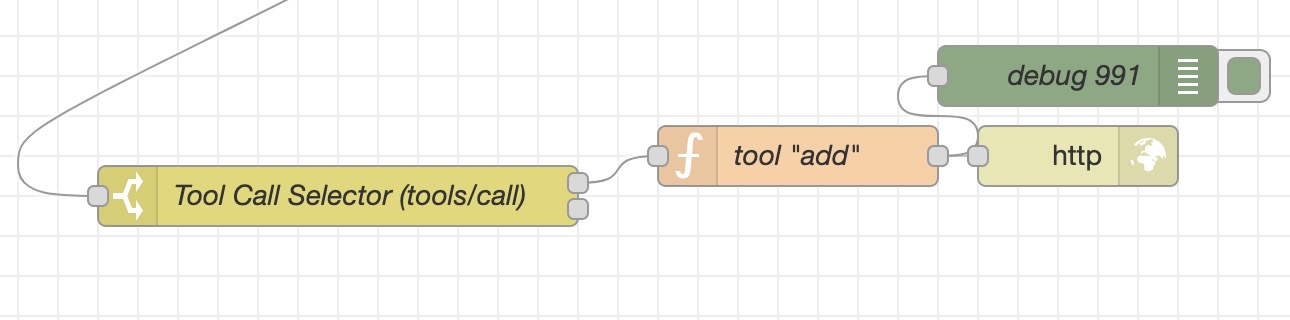
After the MCP client has successfully retrieved the list of available tools using the tools/list
method, it will typically wait for user input or its own internal logic to determine when to invoke a specific tool. The tools/call
method is used for this purpose.
In our example of a simple Math MCP Server, let's consider a user asking the client: "Can you calculate 3 + 14". The MCP client, based on this user message and the available tools it knows about (specifically the "add" tool), will construct a tools/call
request to the server.
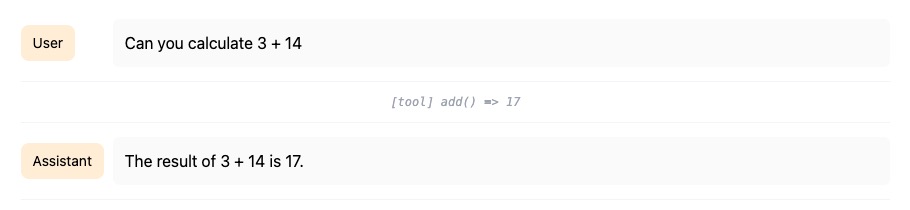
MCP Client Input Example (tools/call
for "add" tool):
{
"method": "tools/call",
"params": {
"name": "add",
"arguments": {
"a": 3,
"b": 14
}
},
"jsonrpc": "2.0",
"id": 2
}
- MCP Server Output Example:
{
"result": {
"content": [
{
"type": "text",
"text": "17"
}
]
},
"jsonrpc": "2.0",
"id": [current message count for the session]
}
- Code Explanation for "tools/list" Function:
msg.result = msg.payload.params.arguments.a + msg.payload.params.arguments.b
let sessions = global.get("sse_sessions") || {};
let session = sessions[msg.req.query.sessionId];
if (session) {
session.messages = session.messages || [];
let message = { "result": { "content": [{ "type": "text", "text": msg.result.toString() }] }, "jsonrpc": "2.0", "id": session.messages.length };
msg.message = message;
session.messages.push({
data: message, // message to send
sent: false // initially unsent
});
global.set("sse_sessions", sessions);
}
msg.res._res.setHeader("Content-Type", "text/plain");
return msg;
- It then performs the addition operation using the
a
andb
arguments provided in the client'stools/call
request. - The function retrieves the current active session using the
sessionId
from the HTTP request. - It constructs a JSON response containing the result of the addition. This response is formatted as a text content element within the
result
object, following the MCP structure. - This constructed response is added to the
session.messages
queue. Thesent
flag is set tofalse
, indicating that this message has not yet been sent to the client via the SSE connection. - The global
sse_sessions
object is updated to reflect the new message in the session's queue. - Finally, the function prepares the HTTP response to the initial
POST
request, setting its payload to "Accepted" and theContent-Type
header totext/plain
. This acknowledges that the server has received thetools/call
request. The actual result of the tool execution is delivered asynchronously through the established SSE connection.
Conclusion: Building Your Remote MCP Server with Node-RED
This comprehensive guide has walked you through the initial steps of building a Remote MCP Server using Node-RED. By leveraging Node-RED's visual programming environment and its HTTP and function nodes, you've learned how to:
- Establish an SSE Endpoint (
/sse
): This crucial endpoint enables persistent, real-time communication from the server to MCP clients, initiating sessions and providing the necessary endpoint information for subsequent client-to-server messages. - Handle Incoming Client Messages (
/sse/message
): This endpoint receivesPOST
requests from clients, validates the session ID to ensure secure communication, and routes messages based on the specified MCP method. - Implement Core MCP Methods: You've built logic to handle fundamental MCP client requests like
initialize
(for server discovery),notifications/initialized
(for client acknowledgment), andtools/list
(for advertising server capabilities). - Enable Tool Invocation (
tools/call
): The server is equipped to receive and process requests to execute specific tools, demonstrated with a simple "add" function, showcasing the server's ability to extend the client's capabilities. - Manage Sessions: The global
sse_sessions
object provides a mechanism to track and manage active client connections, ensuring that messages are correctly associated with the appropriate session. - Utilize Debugging Tools: The guide highlighted the importance of Node-RED's debug nodes and introduced the MCP Inspector as valuable tools for testing and verifying the server's functionality.
By constructing these flows, you've laid a solid foundation for a functional Remote MCP Server. This server can now interact with MCP clients, enabling AI agents to discover and utilize server-side tools to enhance their problem-solving abilities.
The modular nature of Node-RED allows for easy expansion of this server. Future development could involve:
- Implementing more sophisticated tools: Adding a wider range of functionalities that MCP clients can leverage.
- Enhancing session management: Implementing mechanisms for session timeouts, persistent storage, or more complex session states.
- Adding error handling and logging: Improving the robustness and observability of the server.
- Integrating with external services: Connecting the MCP server to other APIs or data sources to further extend its capabilities.
This guide serves as a starting point, empowering you to build increasingly complex and intelligent Remote MCP Servers to facilitate seamless interaction with AI agents within the Model Context Protocol ecosystem.